Flutterのエラー「Non-nullable instance field ‘XX’ must be initialized」の意味と対策について紹介します。
「Non-nullable instance field ‘XX’ must be initialized」の意味
Flutterでデバッグすると「Non-nullable instance field ‘XX’ must be initialized」というエラーが出ることがあります。
例えば、以下のようなコードだと「Non-nullable instance field ‘_id’ must be initialized」というエラーが出ます。
class Todo {
int _id; ← エラー
String _title;
String _description;
String _year;
String _month;
String _day;
int _priority;
Todo(this._title, this._priority, this._year, this._month, this._day, [this._description]); ← エラー
Todo.withId(this._id, this._title, this._priority, this._year, this._month, this._day, [this._description]);
(略)
}
最新版のFlutterでは「Null safety」が導入されたため、変数の値にNullを容易に入れることができなくなりました。
変数にNullを入れるには以下のような記述が追加で必要です。
- 変数をNull許容型で宣言する
- 型名の末尾に?をつけると、その変数はNull許容型となり、Nullを入れることができます。
- (例)String? name;
- 特別な修飾子(?・!・late・required)
【対策方法1】変数宣言時に初期値を与える
以下のように変数の初期値を与えてやることでエラーは消えます。
class Todo {
int _id = 0; ← 修正
String _title;
String _description;
String _year;
String _month;
String _day;
int _priority;
Todo(this._title, this._priority, this._year, this._month, this._day, [this._description]);
Todo.withId(this._id, this._title, this._priority, this._year, this._month, this._day, [this._description]);
(略)
}
【対策方法2】lateで変数宣言
以下のように変数のlateで変数宣言することでもエラーは消えます。
class Todo {
late int _id; ← 修正
String _title;
String _description;
String _year;
String _month;
String _day;
int _priority;
Todo(this._title, this._priority, this._year, this._month, this._day, [this._description]);
Todo.withId(this._id, this._title, this._priority, this._year, this._month, this._day, [this._description]);
(略)
}
【対策方法3】lateで変数宣言
以下のように変数に修飾子?をつけるとエラーは消えます。
class Todo {
late int? _id; ← 修正
String _title;
String _description;
String _year;
String _month;
String _day;
int _priority;
Todo(this._title, this._priority, this._year, this._month, this._day, [this._description]);
Todo.withId(this._id, this._title, this._priority, this._year, this._month, this._day, [this._description]);
(略)
}
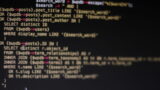
【Flutter入門】iOS、Android、Windowsアプリ開発
FlutterによるiOS、Android、Windowsアプリ開発について入門者向けに紹介します。
コメント